Flutter 中的广播Stream 与 TabBar 导航切换
Android 原生开发中,有 Broadcast 可以用来广播一个 Intent
SDK 也有 EventBus 可以使用
Flutter 中想要广播一个数据,原生可以使用的是 Stream
它与 Future 一样,位于 async
,用来异步使用
例如目前我使用了 TabBar
创建了三个 tab
需要在其他页面异步获取数据后,根据状态,滑动 TabBar
到指定页面
创建
首先创建一个 dart 格式文件,定义文件内容如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import 'dart:async';
class StateSubject { static final StateSubject _instance = StateSubject._();
factory StateSubject() => StateSubject._instance;
StreamController<int> streamController;
StateSubject._() { streamController = StreamController.broadcast(); }
void update(int num) { streamController.sink.add(num); } }
|
在 app 加载时订阅这个 Stream
例如在 initState
中订阅
使用 StateSubject().streamController.stream.listen
,传入一个方法参数进行监听
1 2 3 4 5 6 7 8 9 10 11 12 13
| @override void initState() { super.initState(); controller = TabController(length: 3, vsync: this); StateSubject().streamController.stream.listen((data) { log("listen a data:" + data.toString()); if (data > controller.length || data < 0) { return; } // 设置 TabBar 下标位置 controller.index = data; }); }
|
使用
在任意地方调用即可
1 2 3 4 5 6 7
| // 点击按钮 clickAddBtn() { log("click add btn");
//将首页的tabBar 定位到 index = 1 StateSubject().update(1); }
|
效果
首页:
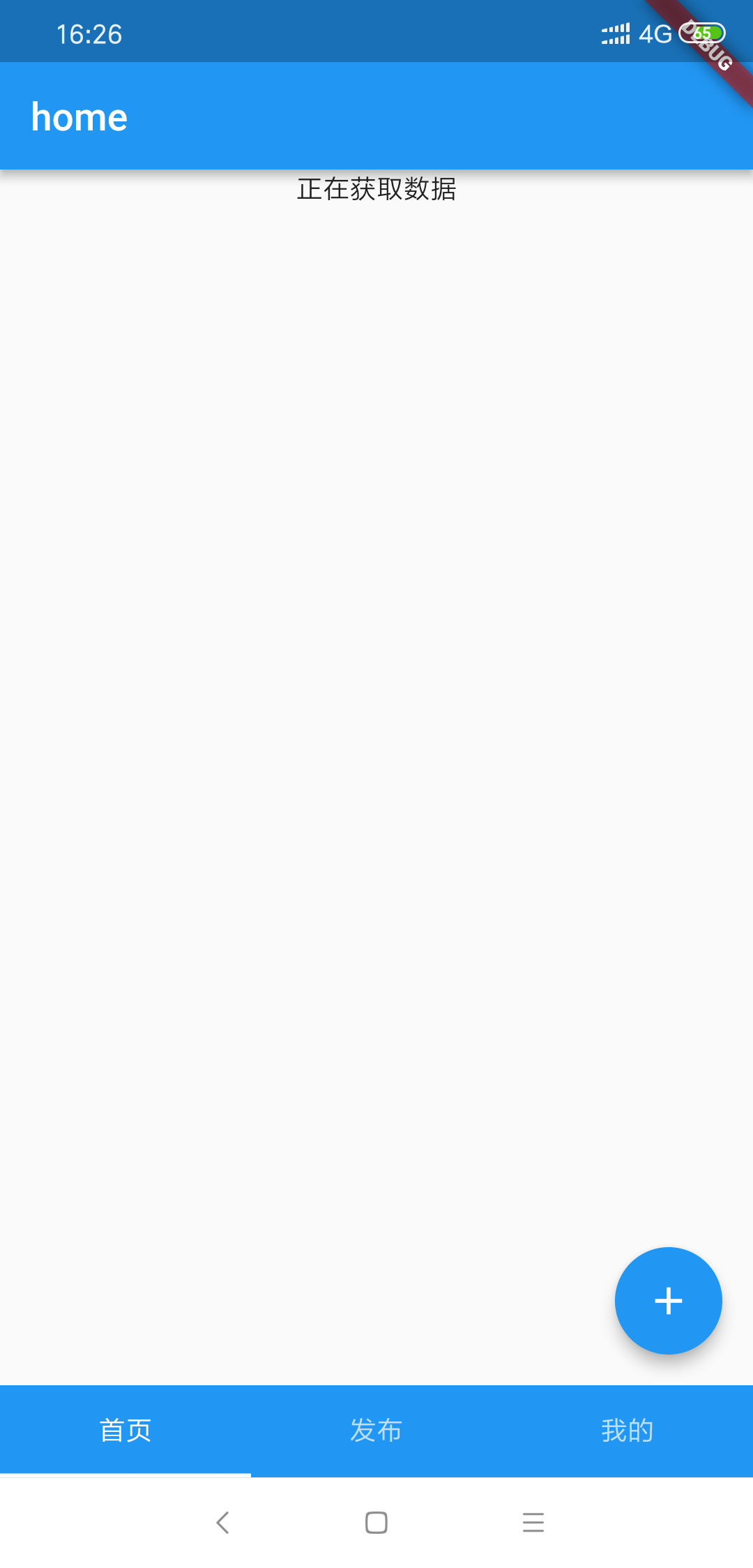
点击按钮后跳到第二页,如果未登陆,跳到第三页要求登录
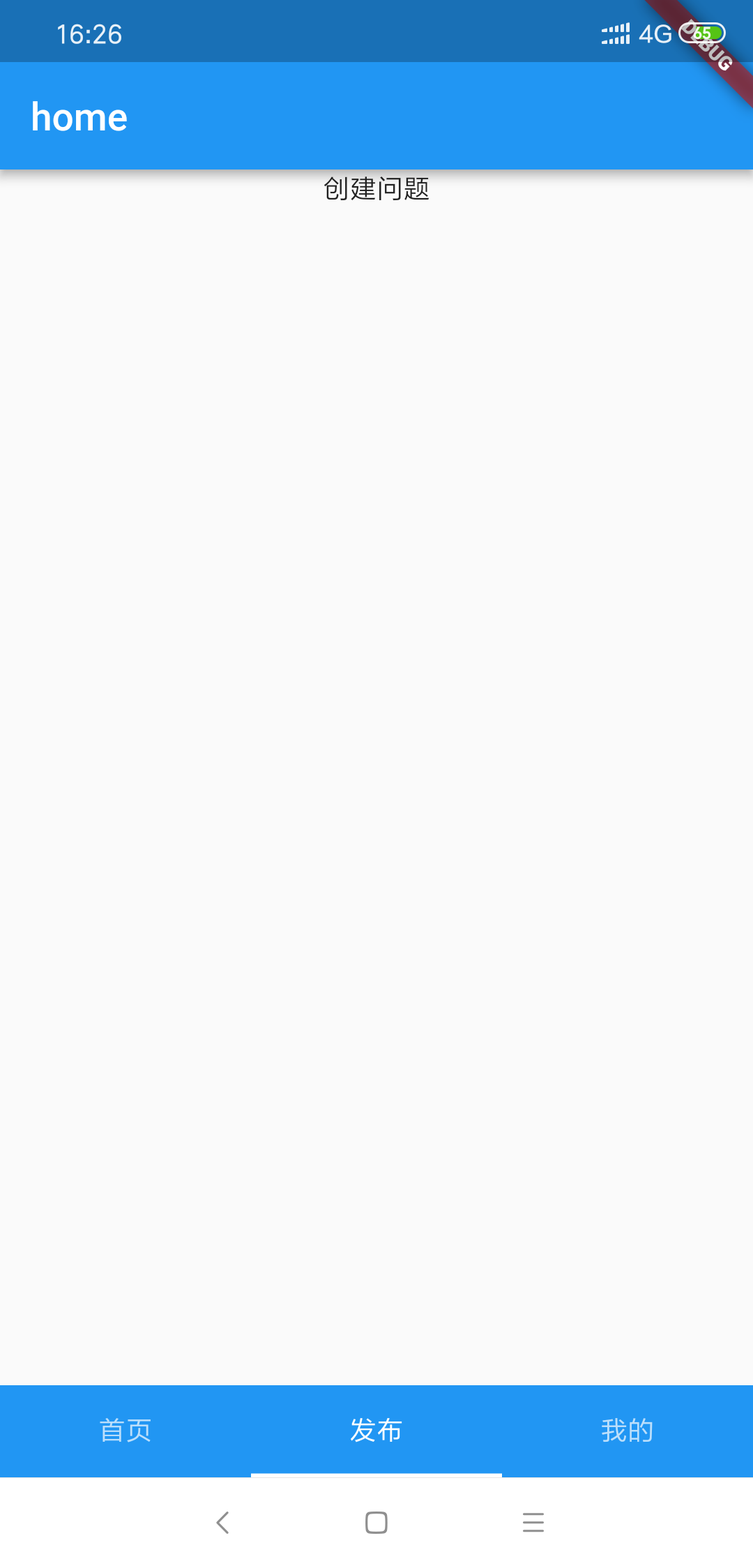
附上TabBarView的使用
定义TabController
首先创建 StatefulWidget
1 2 3 4 5 6 7 8
| class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override _MyHomePageState createState() => _MyHomePageState(); }
|
创建 State
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| class _MyHomePageState extends State<MyHomePage>{ TabController controller;
@override void initState() { // TODO: implement initState super.initState(); controller = TabController(length: 3, vsync: this); }
@override void dispose() { super.dispose(); controller.dispose(); }
@override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Scaffold( body: new TabBarView(controller: controller, children: <Widget>[ new HomePage(), new CreatePage(), new MyPage() ]), bottomNavigationBar: new Material( color: Colors.blue, child: new TabBar(controller: controller, tabs: [ new Tab( text: "首页", ), new Tab( text: "发布", ), new Tab( text: "我的", ) ]), ), ), ); }
}
|
HomePage
CreatePage
MyPage
需要另外创建